当前位置 博文首页 > unity工具人的博客:获取 Image 组件上的Texture纹理,保存成图
using System.Collections;
using System.Collections.Generic;
using System.IO;
using UnityEngine;
using UnityEngine.UI;
public class SavePng : MonoBehaviour
{
[Tooltip("截图存储路径")]
public string path;
public bool capture;
public RawImage rawImage;
Texture2D mtexture;
//保存图像信息,时间戳+像素RGB
private struct ImageData
{
public long timestamp;
public byte[] data;
}
// Start is called before the first frame update
void Start()
{
path = Application.streamingAssetsPath+"/Pictures/";
}
// Update is called once per frame
void Update()
{
if (Input.GetKeyDown(KeyCode.S))
InputSave();
}
//按钮回调
public void InputSave()
{
if (!capture)
{
capture = true;
StartCoroutine(CaptureAndSave02());
Debug.Log("Successd!");
}
}
public IEnumerator CaptureAndSave02()
{
if (capture)
{
//等待屏幕渲染结束后获取屏幕像素信息
yield return new WaitForEndOfFrame();
mtexture = TextureToTexture2D(rawImage.texture);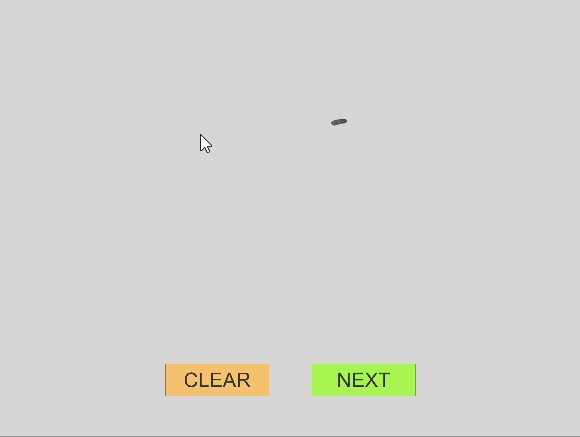
mtexture.Apply();
//将图片信息编码为字节信息
ImageData pack;
pack.data = mtexture.EncodeToPNG();
//保存图片到 你的路径
pack.timestamp = System.DateTimeOffset.UtcNow.ToUnixTimeMilliseconds();
FileStream file = File.Create(path + pack.timestamp + ".png");
file.Write(pack.data, 0, pack.data.Length);
file.Close();
capture = false;
}
}
/// <summary>
/// 运行模式下Texture转换成Texture2D
/// </summary>
/// <param name="texture"></param>
/// <returns></returns>
private Texture2D TextureToTexture2D(Texture texture)
{
Texture2D texture2D = new Texture2D(texture.width, texture.height, TextureFormat.RGBA32, false);
RenderTexture currentRT = RenderTexture.active;
RenderTexture renderTexture = RenderTexture.GetTemporary(texture.width, texture.height, 32);
Graphics.Blit(texture, renderTexture);
RenderTexture.active = renderTexture;
texture2D.ReadPixels(new Rect(0, 0, renderTexture.width, renderTexture.height), 0, 0);
texture2D.Apply();
RenderTexture.active = currentRT;
RenderTexture.ReleaseTemporary(renderTexture);
return texture2D;
}
}
2021年3月28日使用一次
ImageData pack;
pack.data = mtexture.EncodeToPNG();
//保存图片到 你的路径
pack.timestamp = System.DateTimeOffset.UtcNow.ToUnixTimeMilliseconds();
FileStream file = File.Create(path + pack.timestamp + ".png");
file.Write(pack.data, 0, pack.data.Length);
file.Close();
其它:
//从本地读取文件返回byte
byte[] ReadImg(string fileName)
{
FileInfo fileInfo = new FileInfo(Application.dataPath + "/" + fileName);
byte[] buffer = new byte[fileInfo.Length];
using (FileStream fs = fileInfo.OpenRead())
{
fs.Read(buffer, 0, buffer.Length);
}
return buffer;
}
cs